Concepts¶
When you start esc, you will see a status bar and four windows: Stack, History, Commands, and Registers. These windows match up neatly with the important concepts in esc.
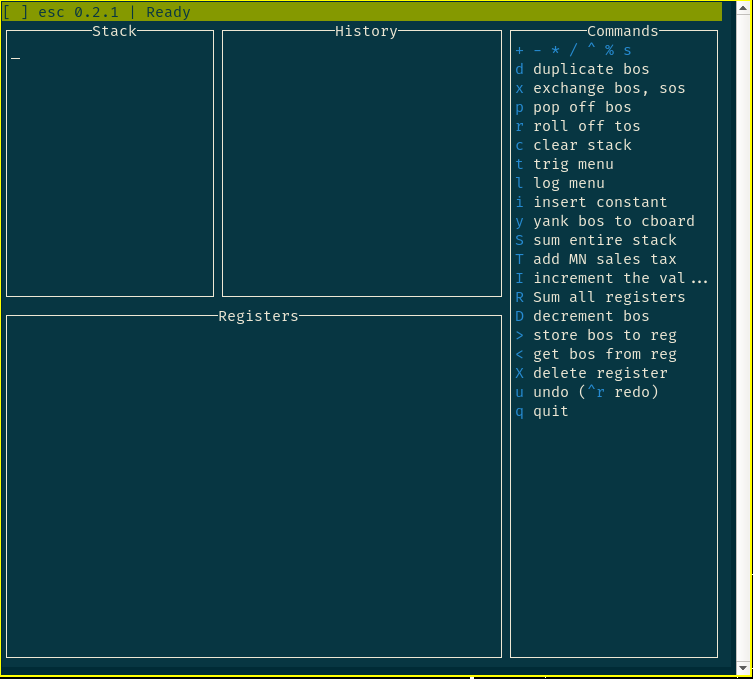
A typical esc window at startup.
Stack¶
The stack contains a series of numbers you are currently working with. Almost all commands follow the pattern of:
- read numbers from the stack;
- do something with them;
- return other numbers to the stack.
As long as no other operation is in progress,
your cursor will be positioned in the Stack window.
To enter a new number (this is called pushing it onto the stack),
simply start typing the number.
The indicator at the left of the status bar will change to [i]
to indicate that you’re inserting a number,
and the numbers will appear in the stack window as you type.
When you’re done typing the number, press Enter or Space;
your cursor will then move to the next line of the stack.
If you make a typo, correct it with Backspace.
(If you’ve already pressed Enter,
an undo will let you edit the number again.)
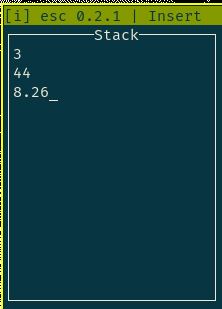
Entering several numbers onto the stack.
You can enter as many numbers as you like this way, at least until the stack fills up. (In esc, the stack size is based on the number of lines allocated for the stack on the screen, which is ordinarily 12. 12 entries should be more than enough for all but the craziest calculations; many RPN-based HP calculators only have four, and that is normally enough.)
Enter negative signs with _ (underscore)
and scientific notation with e
(e.g., 3.66e11
is 3.66 × 10^12 or 366,000,000,000).
Tip
You need only press Enter or Space
between consecutively typed numbers.
If you type a number and then wish to
perform an operation,
you can simply press the key for the operator.
For instance, typing 2 2+
is equivalent to typing 2 2 +
.
Commands¶
Arithmetic operations¶
Eventually you’ll probably get tired of typing in numbers and want to actually perform some operation on the contents of the stack. In the Commands window, you will see a list of operations that you can perform. At the very top are listed the typical arithmetic operations. To perform one of these operations, simply press the associated key. For instance, to perform addition, you press +.
When you perform an operation,
it removes a certain number of entries from the bottom of the stack
(this is called popping them off the stack).
It then uses those values to calculate the result
and pushes the result back onto the bottom of the stack.
For example, if your stack is [1, 2, 3]
and you press the + key to add two numbers,
the 1
is untouched,
while the 2
and 3
are removed from the stack
and the answer, 5
, is pushed on,
so that the stack now has two entries, 1 and 5.
Note
Most operations pop one or two items from the stack and push one answer. However, this is not a requirement; an operator could pop four values and push two back.
You can see how many and what values an operation pops and pushes in its help page.
Stack operations¶
In addition to the arithmetic operations, some stack operations are provided. These don’t calculate anything but allow you to manipulate the contents of the stack.
-
esc.functions.
duplicate
(bos)[source]¶ Duplicate bos into a new stack entry. Useful if you want to hang onto the value for another calculation later.
Other commands¶
In addition to the arithmetic and stack manipulation commands described above, esc defines several special commands.
builtin_stubs.py - stub classes for built-in commands
-
class
esc.builtin_stubs.
StoreRegister
[source]¶ Copy the bottommost value on the stack into a register. Registers store values under a single-letter name until you need them again.
See Registers for more information on registers.
-
class
esc.builtin_stubs.
RetrieveRegister
[source]¶ Copy the value of a register you’ve previously stored to the bottom of the stack. Registers store values under a single-letter name until you need them again.
See Registers for more information on registers.
-
class
esc.builtin_stubs.
DeleteRegister
[source]¶ Remove an existing register from your registers list and destroy its value.
See Registers for more information on registers.
-
class
esc.builtin_stubs.
Undo
[source]¶ Undo the last change made to your stack. Registers are unaffected. (This is a feature, not a bug: a common esc workflow is to reach an answer, then realize you need to go back and do something else with those same numbers. Registers allow you to hold onto your answer while you do so.)
See History for more information on calculation history.
Custom commands¶
In addition to all the commands described above, you may see some other commands in your list at times. These are added by esc plugins.
Getting help on commands¶
esc has a built-in help system you can use to discover what a command does, including the exact effect it would have on your current stack if you ran it. Simply press F1, then the key associated with the command. If you choose a menu, you’ll get a description of the menu, but you can also choose an item from the menu to get specific help on that item.
History¶
esc maintains a complete history of all the numbers you push onto the stack and operations you perform. The operations you execute and brief descriptions of their results are displayed in the History window in the middle of the screen.
If you perform an operation and then want to back up,
simply choose Undo
.
To undo an undo, use Redo
.
Calculation history you can step through is so useful it’s amazing how few calculators offer it.
Registers¶
In addition to placing numbers on the stack, sometimes you might want to keep track of numbers in a slightly more permanent way. In this case, you can store the number to a register.
- To
store to a register
, press >, then type an upper- or lowercase letter to name the register. The bottom item on the stack is copied into the Registers window. - To
retrieve the value of a register
, press <, then type the letter corresponding to the register whose value you want to retrieve. The value is copied into a new item at the bottom of a stack. - To
delete a register
, press X, then type the letter of the register you want to delete. It is removed from the Registers window and its value is lost.
Note
Registers do not participate in the undo/redo history. This is a feature, not a bug: a common esc workflow is to perform some calculation, then realize you needed those numbers again for something. You can store your answer to a register, then undo as needed to get those numbers back.